How to get KPIs and metrics from the Stripe API
In this article we will explain how we get your metrics data from Stripe to display in MinimalDashboard, but if you want to use this data in your own application you can either use our API or follow the instructions below to write your own implementation.
Stripe is a global payment platform enabling businesses to accept payments online, manage transactions, and build financial infrastructure efficiently with a best in class developer API. You can find their documentation here: Stripe API Documentation.
Contents
- Authentication
- Endpoints
- How to get total revenue from Stripe
- How to get total revenue from Stripe by product or price
- How to get monthly recurring revenue from Stripe
Authentication
For authentication you need a Stripe API key which you can get from the Dashboard > Developers
page and then selecting the API keys tab
.
Add this key as the Bearer
to each request you’re making.
Endpoints
The payment_intents
endpoint (Documentation) is the ultimate list of payments in your account. The older charges
endpoint should not be used anymore as Stripe is switching to payment intents going forward.
Stripe Paging
Stripe utilizes cursor based paging. To fetch the next page simply specify a query parameter starting_after
with the latest (largest) id
of the previous response.
How to get total revenue from Stripe
In order to calculate the total revenue for a given date period simply send a GET request to this endpoint specifying the date range.
https://api.stripe.com/v1/payment_intents
// filter by date range
?created[gte]=1725188242 // Unix Seconds Timestamp
&created[lt]=1726491443 // Unix Seconds Timestamp
This will result in a list of payment intent objects.
{
"object": "list",
"data": [
{
"id": "pi_3PzcCyI5q0oROU991WkRixe7",
"amount": 50400,
"amount_received": 50400,
"currency": "usd",
"created": 1726482664,
"status": "succeeded",
},
],
"has_more": false,
"url": "/v1/payment_intents"
}
To calculate your total revenue you can sum up the amount values which represent cents.
Note: the amount is in the transaction currency, which might differ from your payout currency. MinimalDashboard asks you to specify which currency you want to display in your dashboard and convert the values to that currency.
How to get total revenue from Stripe by product or price
In order to calculate the total revenue for a specific product or price in a given date period simply send a GET request to this endpoint specifying the date range and requesting the invoice line items to be expanded.
https://api.stripe.com/v1/payment_intents
// filter by date range
?created[gte]=1725188242 // Unix Seconds Timestamp
&created[lt]=1726491443 // Unix Seconds Timestamp
// request the line items
&expand[]=data.invoice.lines
This will result in a list of payment intent objects with the invoice information which contains product and plan information you can use to filter.
{
"object": "list",
"data": [
{
"id": "pi_123",
"amount": 50400,
"amount_received": 50400,
"currency": "usd",
"created": 1726482664,
"invoice": {
"lines": {
"data": [
{
"id": "il_123",
"amount": 147600,
"price": {
"id": "price_123",
"product": "prod_123",
"type": "recurring",
},
},
...
],
"has_more": false,
"total_count": 1,
"url": "/v1/invoices/in_123/lines"
},
},
"status": "succeeded",
},
],
"has_more": false,
"url": "/v1/payment_intents"
}
But an invoice is only (automatically) generated for subscriptions. To get product and price information for one-time products, you need to request the checkout session associated with the payment intent which you can then expand the line items for.
https://api.stripe.com/v1/checkout/sessions
// Use payment intent id from the above response
?payment_intent=pi_123
// request the line items
&expand[]=data.line_items
This will result in the following response:
{
"object": "list",
"data": [
{
"id": "cs_123",
"line_items": {
"object": "list",
"data": [
{
"id": "li_123",
"amount_discount": 0,
"amount_subtotal": 50400,
"amount_tax": 0,
"amount_total": 50400,
"currency": "usd",
"price": {
"id": "price_123",
"product": "prod_123",
"type": "one_time",
},
"quantity": 12
}
],
"has_more": false,
"url": "/v1/checkout/sessions/cs_123/line_items"
},
"status": "complete",
}
],
"has_more": false,
"url": "/v1/checkout/sessions"
}
Note that the line item schema for payment_intent
and checkout/sessions
is similar but not identical. The amount is provided in amount
for one and in amount_total
for the other.
To calculate total revenue for a specific price or product, first filter by the price or product id then sum up the amount
(or amount_total
) to find the final amount.
Note: as above the amount is in the transaction currency, which might differ from your payout currency. MinimalDashboard asks you to specify which currency you want to display in your dashboard and convert the values to that currency.
How to get monthly recurring revenue from Stripe
In order to calculate the total revenue for a specific product or price in a given date period send a GET request to this endpoint specifying the date range and requesting the invoice line items to be expanded.
https://api.stripe.com/v1/payment_intents
// filter by date range
?created[gte]=1725188242 // Unix Seconds Timestamp
&created[lt]=1726491443 // Unix Seconds Timestamp
// request the line items
&expand[]=data.invoice.lines
This will result in a list of payment intent objects with the invoice information which contains whether a price line item is recurring.
{
"object": "list",
"data": [
{
"id": "pi_123",
"amount": 50400,
"amount_received": 50400,
"currency": "usd",
"created": 1726482664,
"invoice": {
"lines": {
"data": [
{
"id": "il_123",
"amount": 147600,
"price": {
"id": "price_123",
"product": "prod_123",
**"type": "recurring",**
},
},
...
],
"has_more": false,
"total_count": 1,
"url": "/v1/invoices/in_123/lines"
},
},
"status": "succeeded",
},
],
"has_more": false,
"url": "/v1/payment_intents"
}
For recurring revenue (i.e. subscriptions) this request is sufficient. Filter out any line items that are not type == recurring
and sum up the amount
value.
Note: as above the amount is in the transaction currency, which might differ from your payout currency. MinimalDashboard asks you to specify which currency you want to display in your dashboard and convert the values to that currency.
Looking for more?
Have a look at our API knowledge base with many more services...
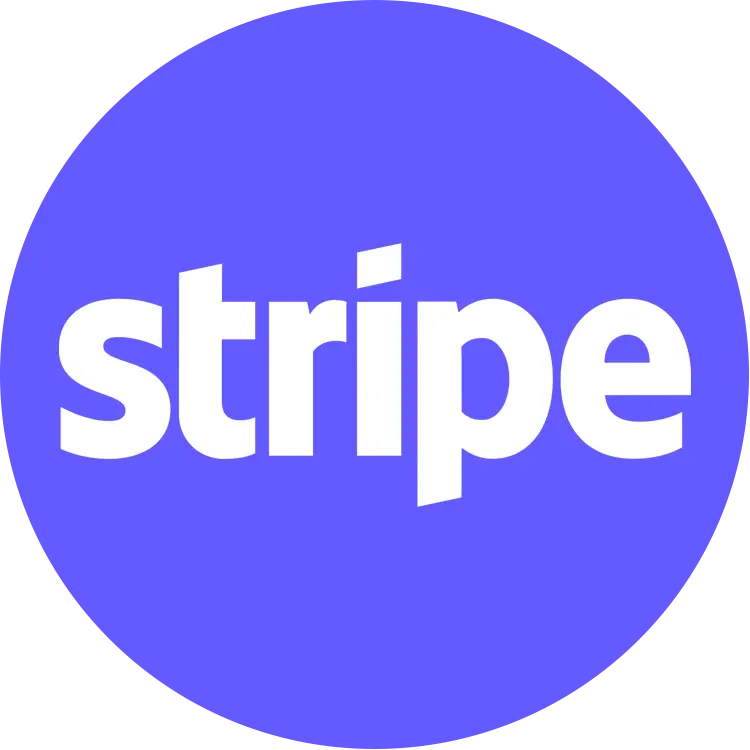
Stripe
Payments
If you'd like to have a ready-made dashboard with all the metrics from Stripe instead, you can create one with MinimalDashboard in a few clicks.
Create dashboards to show
- Revenue
- Recurring Revenue
- Non-Recurring Revenue
- New Subscriptions